Welcome to Bot!Battle!
See how awesome we are!!
Bot!Battle! is an application designed to support and encourage students to gain an early enthusiasm for computer science and programming. Students will write small “bots”, or simple programs that are designed to play a game. Bot!Battle! will host tournaments in which the students’ bots will compete for the title of most awesome bot. In addition, students will be able to test their bots, both against themselves or against another bot, via a publically accessible portal where they can watch their bots play the game. Also, Bot!Battle! is extensible in that it allows new games to be developed and then uploaded for use in new tournaments.
Try our first game for the Bot!Battle! system
Save The Island
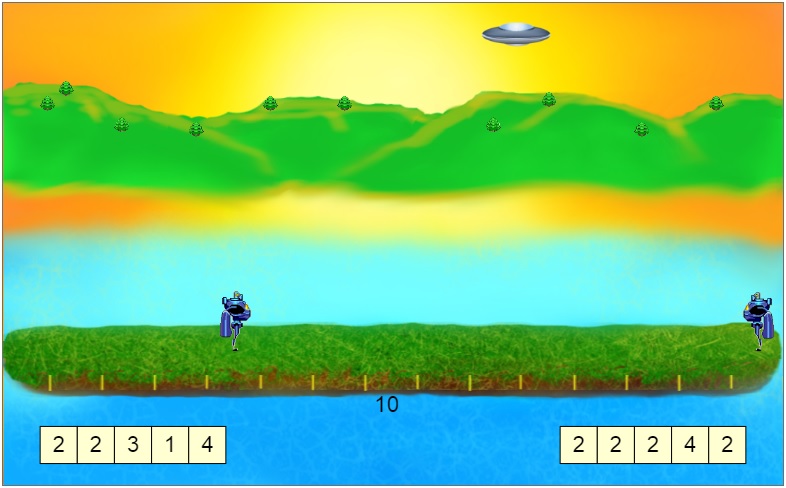
To get started make a bot and then go to the TestArena and try it out!
Here is a sample bot in Java
import java.util.Scanner; public class IslandBattleBot1 { public static int player; public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String board; // This will read in moves while our // GameManager sends your bot updates while(scanner.hasNextLine()){ board = scanner.nextLine(); System.err.println(board); // This will send debug data of your choice to the TestArena! System.out.println(getMove(board)); // This will send our GameManager your bots move! } scanner.close(); } // This is a sample board for Island Battle // 1;22314;000010000000002 public static String getMove(String board) { String move = ""; String[] components = board.split(";"); String playerNum = components[0]; String tiles = components[1]; String island = components[2]; int distance = island.indexOf("2") - island.indexOf("1"); for (int i = 0; i < tiles.length(); i++) { if(Integer.parseInt(tiles.substring(i, i+1)) == distance) { move = "attack;" + tiles.substring(i, i+1); break; } else if( Integer.parseInt(tiles.substring(i, i+1)) < distance ) { move = "move;" + tiles.substring(i, i+1); } } if(move.equals("")){ move = "retreat;" + tiles.substring(0, 1); } return move; } }